- Matrix’s elements sum (calls upon a function that calculates elements sum)
- Maximal value in every row of a matrix (calls upon a function that finds the biggest element in a flow)
Matrix mat is declared in a way of two dimensional array (2D array):
float mat[MAXROW][MAXCOL];
Variables nrRow and nrCol store matrix’s dimensions provided by user. The image below shows you this in a visual way. Click on the image to enlarge it.
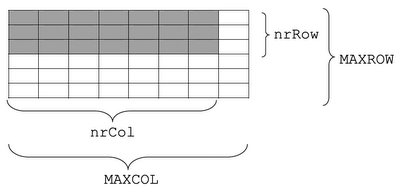
Memory of a computer allocates MAXROW * MAXCOL * sizeof(float) byte. Matrix’s rows follow one after another, in a way where every next row is placed right after the previous one; and so on. Picture below describes this perfectly. Click on the image to enlarge it.
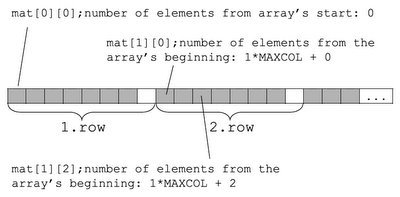
Generally speaking, for mat[i][j] – number of elements from the beginning of an array is: i * MAXCOL + j
#include <stdio.h>
#define MAXROW 100
#define MAXCOL 100
float max( int len, float *p ) {
// OR: float max( int len, float p[] )
float res;
int i;
printf("\nIn function max :");
printf("\nRow’s beginning address
in memory : %ld", p);
res = p[0];
for( i=1; i<len; i++ )
if( p[i] > res )
res = p[i];
return res;
}
float sumEl(int nrRow,int nrCol,
int maxCol,float *mat) {
int i, j;
float sum;
printf("\nIn function sumEl:");
printf("\nMatrix’s beginning address
in memory: %ld", mat);
printf("\nBegin. of 2nd row’s addr.
in mem.: %ld", &mat[maxCol]);
printf("\nBegin. of 3rd row’s addr.
in mem.: %ld", &mat[2* maxCol]);
sum = 0.0;
for( i=0; i<nrRow; i++ )
for( j=0; j<nrCol; j++ )
sum += mat[i*maxCol + j];
// or: sum += *(mat + i*maxCol + j)
return sum;
}
int main(void) {
int row, col, i, j;
float add, maxRow, mat[MAXROW][MAXCOL];
printf("\nInput nr. of rows and columns:");
scanf("%d %d", &row, &col );
printf("\nIn function main");
printf("\nMatrix’s beginning address
in memory: %ld", mat);
printf("\nBegin. of 2nd row’s
addr.: %ld\n\n", &mat[1][0]);
printf("\nBegin. of 3rd row’s
addr.: %ld\n\n", &mat[2][0]);
for( i=0; i<row; i++ )
for( j=0; j<col; j++ ) {
printf("Input element[%d,%d]:",i,j);
scanf("%f", &mat[i][j] );
}
//calculates elements sum in matrix
add=sumEl(row,col,MAXCOL,(float *) mat);
printf("\n\nSum of matrix
elements is %f", add );
//prints maximal value of every mat. row
for(i=0;i<row;i++) {
maxRow = max(col, &mat[i][0]);
// or: max(col, mat+i*MAXCOL)
printf("\nBiggest element in
row %d is %f\n",i,maxRow);
}
}
Example of program’s execution:
Input nr. of rows and columns: 3 2
In function main :
Matrix’s beginning address in memory : 1205032
Begin. of 2nd row’s addr. : 1205432
Begin. of 3rd row’s addr. : 1205832
3rd:
1205432 = 125032 + 1 * MAXCOL * sizeof(float)
= 125032 + 400;
1205832 = 125032 + 2 * MAXCOL * sizeof(float)
= 125032 + 800;
Input element [0,0]: 2
Input element [0,1]: 3
Input element [1,0]: 4
Input element [1,1]: 3
Input element [2,0]: 1
Input element [2,1]: 5
In function sumEl:
Matrix’s beginning address in memory: 1205032
Begin. of 2nd row’s addr. in mem.: 1205432
Begin. of 3rd row’s addr. in mem.: 1205832
Sum of matrix elements is 18.000000
In function max:
Row’s beginning address in memory: 1205032
Biggest element in row 0 is 3.000000
In function max:
Row’s beginning address in memory: 1205432
Biggest element in row 1 is 4.000000
In function max:
Row’s beginning address in memory: 1205832
Biggest element in row 2 is 5.000000
Example:
Write your own C function which returns flow of matrix row’s maximal values.
void maxFlow(float *mat,int nrRow,int nrCol,
int maxCol,float *flow) {
//OR: void maxFlow(float mat[], int nrRow,
int nrCol,int maxCol,float flow[])
int i, j;
for (i = 0; i < nrRow; i++) {
flow[i] = mat[i * maxCol];
for(j = 1; j < nrCol; j++)
if (mat[i*maxCol+j] > flow[i])
flow[i]=mat[i*maxCol+j];
}
}
How can I get lessons 1-14 I can't find a link?
the links are in a sidebar (or if you are using IE, below this comment box)
Great work, just keep going. When it's complete i'll send all beginers to this site.
I hope you keep this up.. the lessons are pretty hard to understand but when you keep at it you can do it.
For the future, I hope to learn how to get my program to communicate with other windows apps and im sure this guide will be the best place to start.
Tnx for your efforts!!
So, has the ardor faded away at the glimpse of a hardship?
Thank you, I love these example so much, please make lots more, cheers
nice lessons i love this site
Please update this with more lessons and as BBX said. A tutorial (how-to) guide on how to communicate w/ other windows programs would be awesome.
keep up the good work theyre great
U R a SUPER-HUGE geek.. jesus. ... and I am wayyy jealous of your ninja-like C skills.
I love it very much...
Thank
Ya !! This is an interesting website that i have ever searched
I like its tutorials very much..
I will be thankful to you if u please send me latters tutorials (news letters) on my e-mail id
Thanks
Shrikant Divedi
BCA (IGNOU University)